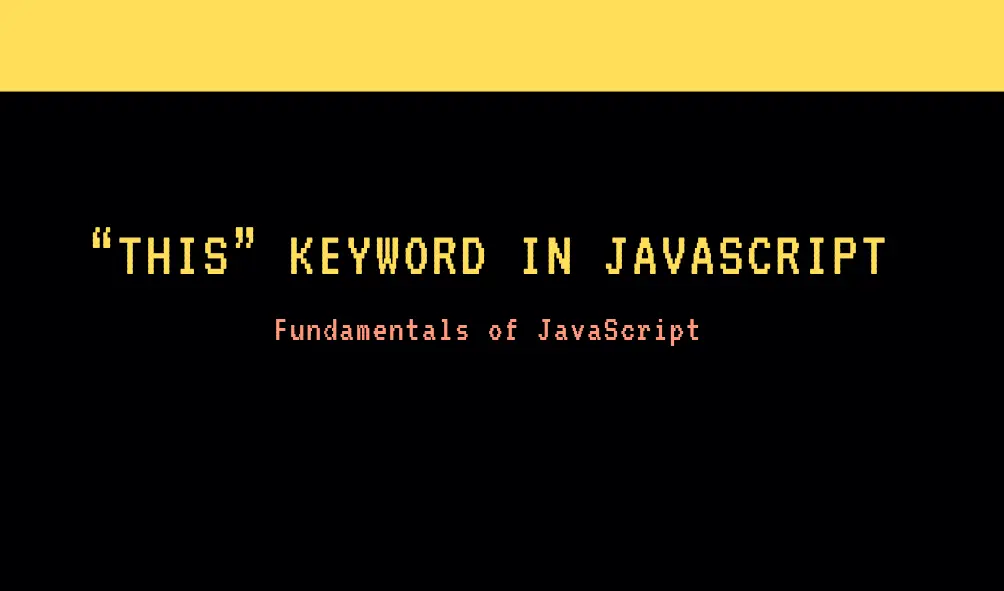
Understanding "this" in JavaScript
8. Understanding “this” keyword
A. What is “This” In JavaScript
In JavaScript, the this
keyword is a special identifier that refers to the context in which a function is executed. The value of this
is determined by how a function is called, and it can vary depending on the invocation context.
Global Context
Outside of any function, this
refers to the global object in non-strict mode. In a web browser, the global object is window
.
console.log(this === window); // true
Function Context
In a regular function call, this
refers to the global object in non-strict mode, but it is undefined
in strict mode.
function greet() {
return this;
}
console.log(greet() === window); // true in non-strict mode, false in strict mode
Method Context
When a function is called as a method of an object, this
refers to the object on which the method is called.
let person = {
name: 'John',
greet: function () {
return 'Hello, ' + this.name;
},
};
console.log(person.greet()); // Hello, John
Constructor Context
When a function is used as a constructor with the new
keyword, this
refers to the newly created object.
function Person(name) {
this.name = name;
}
let john = new Person('John');
console.log(john.name); // John
Event Handler Context
In event handler functions, this
refers to the element that triggered the event.
<button id="myButton">Click Me</button>
<script>
document.getElementById('myButton').addEventListener('click', function () {
console.log(this.id); // myButton
});
</script>
Arrow Function Context
Arrow functions do not have their own this
context. They inherit this
from the enclosing lexical scope.
let obj = {
foo: function () {
setTimeout(() => {
console.log(this === obj); // true
}, 100);
},
};
obj.foo();
Choosing the Right Context
Understanding how this
works is important for choosing the right context in different scenarios:
- Global Scope: Use
this
cautiously in the global scope to avoid unexpected behavior. - Function Context: Be aware of
this
behavior in regular function calls, especially in strict mode. - Method Context: Use
this
in methods to access properties of the object on which the method is called. - Constructor Context: Use
this
to set properties on newly created objects within constructors. - Event Handlers: Use
this
to refer to the element that triggered the event in event handler functions. - Arrow Functions: Be cautious when using arrow functions as methods or event handlers, as they do not have their own
this
context.
Conclusion
this
in JavaScript is a powerful and versatile mechanism for dynamically determining the execution context of functions. Understanding how this
works in different scenarios is essential for writing clear, concise, and predictable JavaScript code. It allows developers to access and manipulate data within the appropriate context, enabling the creation of more flexible and maintainable applications.
B. Call and Bind
In JavaScript, call()
and bind()
are methods available on functions that allow you to control the this
context and pass arguments to functions. These methods are part of the Function prototype and are essential for managing the execution context of functions, especially in an object-oriented or event-driven environment.
Function.prototype.call()
The call()
method calls a function with a specified this
value and arguments provided individually. It immediately invokes the function with the given context and arguments.
Syntax
func.call(thisArg, arg1, arg2, ...);
Parameters
- thisArg: The value to be used as
this
when the function is called. It can be any object ornull
/undefined
(defaulting to the global object in non-strict mode). - arg1, arg2, …: Arguments to be passed to the function.
Example
function greet(greeting, punctuation) {
console.log(greeting + ', ' + this.name + punctuation);
}
let person = {
name: 'Alice',
};
greet.call(person, 'Hello', '!'); // Hello, Alice!
In this example:
- The
greet
function is called withthis
set toperson
. - The arguments
'Hello'
and'!'
are passed individually.
Function.prototype.bind()
The bind()
method creates a new function that, when called, has its this
keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called. Unlike call()
, bind()
does not immediately invoke the function but returns a new function with the bound context.
Syntax
let boundFunc = func.bind(thisArg, arg1, arg2, ...);
Parameters
- thisArg: The value to be used as
this
when the function is called. - arg1, arg2, …: Arguments to prepend to arguments provided to the bound function when invoking it.
Example
let person = {
name: 'Bob',
greet: function (greeting, punctuation) {
console.log(greeting + ', ' + this.name + punctuation);
},
};
let greetBob = person.greet.bind(person, 'Hi');
greetBob('!!!'); // Hi, Bob!!!
In this example:
person.greet.bind(person, 'Hi')
creates a new functiongreetBob
withthis
set toperson
and the first argument preset to'Hi'
.- When
greetBob
is called with'!!!'
, it produces the outputHi, Bob!!!
.
Comparison Between call()
and bind()
- Invocation:
call()
: Immediately invokes the function with the specifiedthis
value and arguments.bind()
: Returns a new function with the specifiedthis
value and arguments bound, which can be invoked later.
- Usage:
call()
: Useful when you need to invoke a function once with a specific context.bind()
: Useful when you need to create a function with a fixed context that can be reused.
Practical Use Cases
Using call()
for Method Borrowing
let person1 = { name: 'Charlie' };
let person2 = { name: 'Dave' };
function sayName() {
console.log(this.name);
}
sayName.call(person1); // Charlie
sayName.call(person2); // Dave
Using bind()
for Event Handlers
function Button(label) {
this.label = label;
this.click = this.click.bind(this);
}
Button.prototype.click = function () {
console.log('Button ' + this.label + ' clicked');
};
let button1 = new Button('Submit');
document.getElementById('submit-btn').addEventListener('click', button1.click);
// Button Submit clicked
In this example, bind()
is used to ensure that this
inside the click
method always refers to the Button
instance, even when the method is used as an event handler.
Conclusion
Understanding call()
and bind()
is crucial for effectively managing function contexts in JavaScript. call()
allows for immediate function invocation with a specific this
context, while bind()
creates a new function with a bound context, useful for scenarios where functions need to be invoked later with a fixed this
value. These methods are powerful tools for writing flexible and reusable JavaScript code.