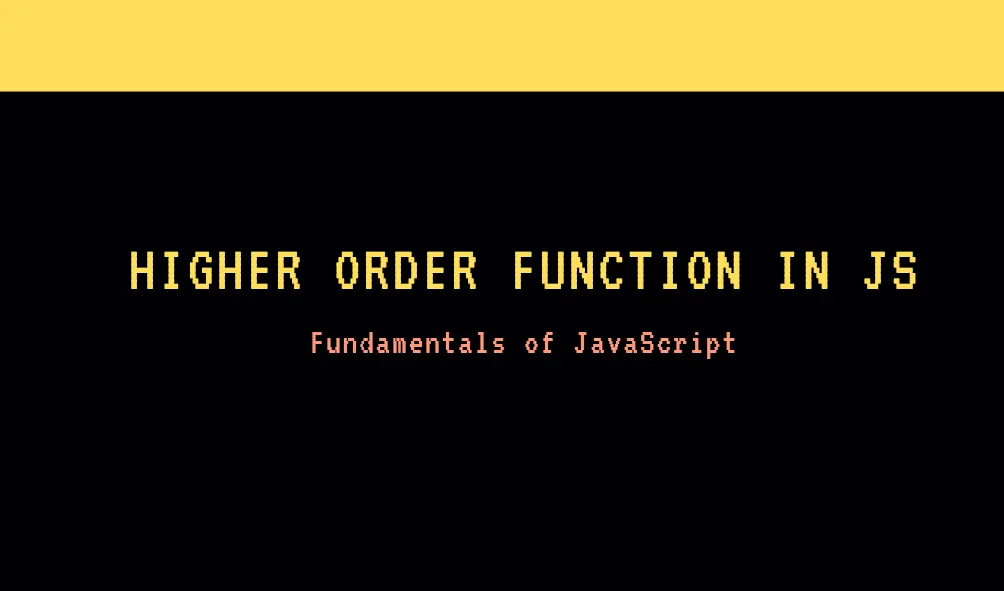
Understanding Higher Order Functions in JavaScript
6. Higher order functions
In JavaScript, higher-order functions are functions that can accept other functions as arguments and/or return functions as their result. Higher-order functions are a powerful feature of the language, enabling functional programming paradigms such as composition, currying, and abstraction of control flow.
Characteristics of Higher-Order Functions:
- Accepting Functions as Arguments:
- Higher-order functions can take other functions as arguments, allowing for dynamic behavior and customization.
- Returning Functions:
- Higher-order functions can return new functions as their result, allowing for function composition and abstraction.
Examples of Higher-Order Functions:
1. Function as an Argument:
function modifyArray(arr, modifierFunction) {
return arr.map(modifierFunction);
}
function double(num) {
return num * 2;
}
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = modifyArray(numbers, double);
console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
In this example, modifyArray
is a higher-order function that accepts an array and a modifier function. It applies the modifier function to each element of the array using Array.map
.
2. Function as a Return Value:
function greet(greeting) {
return function (name) {
console.log(greeting + ', ' + name + '!');
};
}
const greetHello = greet('Hello');
greetHello('John'); // Output: Hello, John!
In this example, greet
is a higher-order function that returns a new function. The returned function (closure) retains access to the greeting
parameter of its parent function.
3. Callback Functions:
function fetchData(url, callback) {
// Simulate fetching data from the URL
const data = { name: 'John', age: 30 };
setTimeout(() => {
callback(data);
}, 1000);
}
function processData(data) {
console.log('Processed data:', data);
}
fetchData('https://example.com/data', processData);
In this example, fetchData
is a higher-order function that accepts a callback function to be invoked once the data is fetched asynchronously.
Benefits of Higher-Order Functions:
- Abstraction and Reusability:
- Higher-order functions promote code abstraction by separating concerns and allowing for reusable function logic.
- Flexibility:
- Higher-order functions enable flexible and dynamic behavior, as functions can be passed as arguments or returned as values based on runtime conditions.
- Functional Composition:
- Higher-order functions facilitate functional composition, allowing developers to combine smaller functions to create more complex behaviors.
- Declarative Programming:
- Higher-order functions promote a declarative programming style, focusing on what needs to be done rather than how it should be done.
Built In Higher Order Functions
1. map()
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map((num) => num * num);
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
The map()
method applies a function to each element of an array and returns a new array with the results.
2. filter()
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter((num) => num % 2 === 0);
console.log(evenNumbers); // Output: [2, 4]
The filter()
method creates a new array with all elements that pass the test implemented by the provided function.
3. reduce()
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log(sum); // Output: 15
The reduce()
method executes a reducer function on each element of the array, resulting in a single output value.
4. forEach()
const numbers = [1, 2, 3, 4, 5];
numbers.forEach((num) => console.log(num * 2));
The forEach()
method executes a provided function once for each array element.
5. sort()
const fruits = ['Banana', 'Orange', 'Apple', 'Mango'];
fruits.sort();
console.log(fruits); // Output: ["Apple", "Banana", "Mango", "Orange"]
The sort()
method sorts the elements of an array in place and returns the sorted array.
6. find()
const numbers = [1, 2, 3, 4, 5];
const foundNumber = numbers.find((num) => num > 3);
console.log(foundNumber); // Output: 4
The find()
method returns the value of the first element in the array that satisfies the provided testing function.
7. some()
const numbers = [1, 2, 3, 4, 5];
const hasEvenNumber = numbers.some((num) => num % 2 === 0);
console.log(hasEvenNumber); // Output: true
The some()
method tests whether at least one element in the array passes the test implemented by the provided function.
8. every()
const numbers = [1, 2, 3, 4, 5];
const allPositive = numbers.every((num) => num > 0);
console.log(allPositive); // Output: true
The every()
method tests whether all elements in the array pass the test implemented by the provided function.
These are just a few examples of built-in higher-order functions in JavaScript. Each of these functions provides powerful tools for manipulating arrays and performing common operations in a functional and expressive manner.