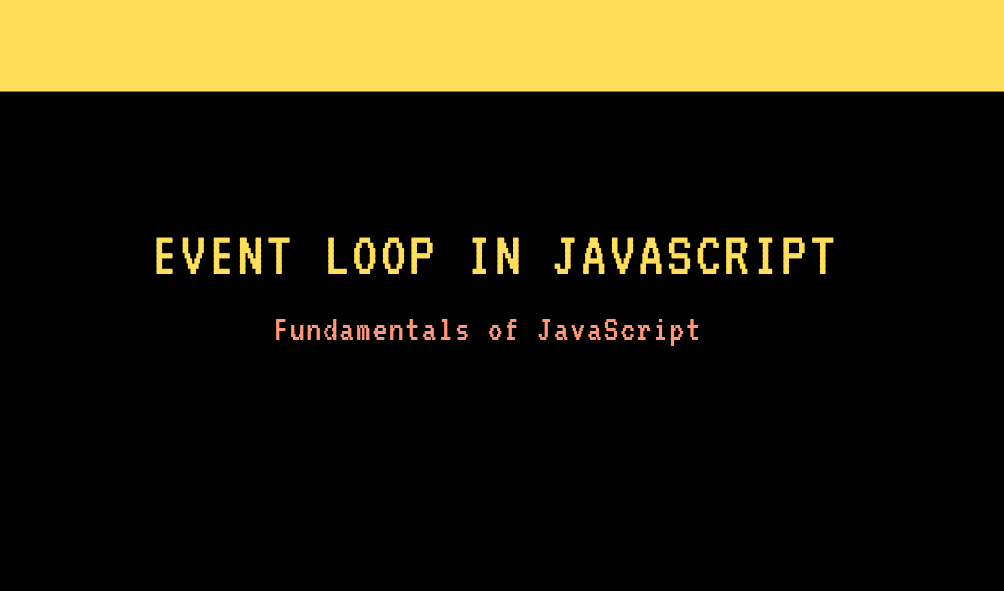
Mastering the JavaScript Event Loop
11. Event Loop
The event loop is a fundamental concept in JavaScript that governs the execution model of asynchronous code in the browser environment. Understanding how the browser uses the event loop is essential for writing efficient and responsive web applications.
Event Loop Overview
The event loop is responsible for managing the execution of asynchronous tasks, such as timers, network requests, and event handlers, in the browser environment. It ensures that tasks are executed in a non-blocking manner, allowing the browser to remain responsive even while performing time-consuming operations.
Execution Phases
The event loop operates in a continuous loop, processing tasks in multiple phases:
- Task Queue:
- Asynchronous tasks, such as timer callbacks, network request responses, and DOM events, are placed into task queues (e.g., the callback queue or microtask queue) once they are completed.
- Tasks in the task queues are processed in subsequent phases of the event loop.
- Execution Stack:
- The JavaScript engine maintains an execution stack, which contains synchronous code that is currently being executed.
- When a function is invoked, it is pushed onto the execution stack. When the function completes, it is popped off the stack.
- Event Loop:
- The event loop continuously checks if the execution stack is empty and if there are any tasks in the task queues.
- If the execution stack is empty and there are tasks in the task queues, the event loop picks a task from one of the queues and pushes it onto the execution stack for execution.
- Once the task is executed, the event loop moves to the next iteration, checking again for tasks in the queues.
Example Scenario
Let’s consider an example scenario to illustrate how the event loop operates:
console.log('Start');
setTimeout(() => {
console.log('Timeout callback');
}, 0);
Promise.resolve().then(() => {
console.log('Promise resolved');
});
console.log('End');
In this example:
- The code starts executing synchronously. “Start” and “End” are logged to the console.
setTimeout()
schedules the timeout callback to be executed after 0 milliseconds. This callback is placed into the timer task queue.Promise.resolve().then()
schedules a microtask to be executed as soon as possible. This microtask is placed into the microtask queue.- The synchronous execution completes, and the event loop starts processing tasks.
- The microtask queue is processed first, executing the microtask callback (
Promise resolved
). Microtasks have higher priority than tasks in the callback queue. - After processing microtasks, the event loop moves to the callback queue and executes the timeout callback (
Timeout callback
).
Conclusion
The event loop is a crucial component of JavaScript’s asynchronous execution model in the browser environment. By efficiently managing the execution of asynchronous tasks, the event loop ensures that web applications remain responsive and performant, even when dealing with time-consuming operations. Understanding how the event loop operates is essential for writing efficient and scalable JavaScript code for web development.