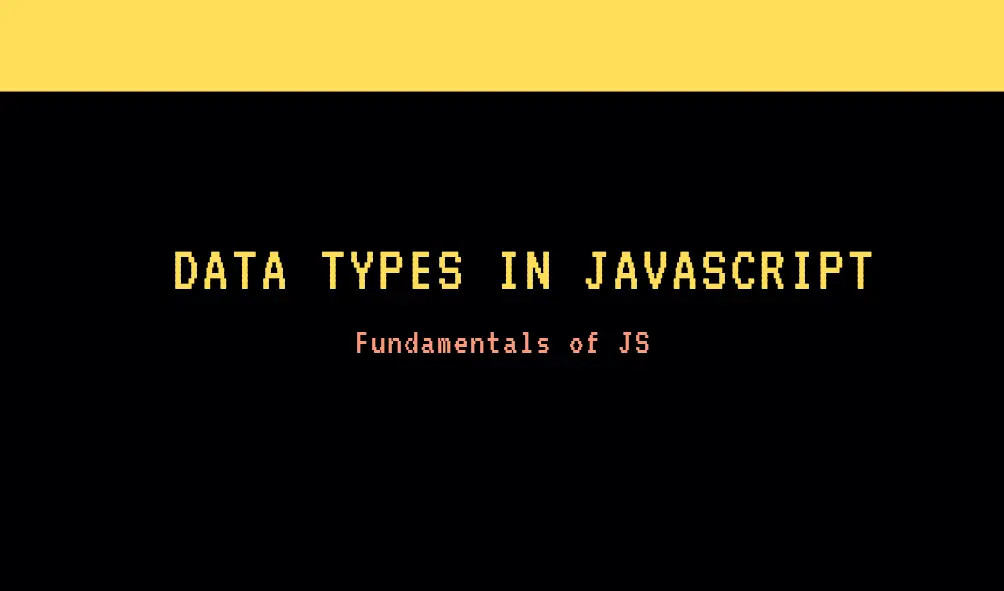
Understanding Data Types in JavaScript
1. Data Types
Primitive Types
Primitive types are the most basic data types in JavaScript. They are immutable, meaning their values cannot be changed once created. Instead, any operation that appears to modify a primitive value actually creates a new value.
Immutability
Primitive values cannot be altered directly.
Assignment and Copying
When a primitive value is assigned to a variable or passed to a function, a copy of the value is created.
let a = 10;
let b = a; // b is a copy of a
b = 20;
console.log(a); // 10
console.log(b); // 20
1. Number
Represents numeric values, including integers and floating-point numbers.
let num = 42;
let pi = 3.14;
2. String
Represents a sequence of characters.
let greeting = 'Hello, world!';
3. Boolean
Represents a logical entity with two values: true
or false
let isJavaScriptFun = true;
4. Undefined
Represents a variable that has been declared but not assigned a value.
let someVariable;
console.log(someVariable); // undefined
5. Null
Represents the intentional absence of any object value.
let emptyValue = null;
6. Symbol
Represents a unique and immutable identifier.
let sym = Symbol('description');
7. BigInt
let bigIntValue = 1234567890123456789012345678901234567890n;
Reference Type
Reference types, also known as complex types, are objects. These types can store collections of values and more complex entities. Objects, arrays, and functions are reference types.
Mutability
The values of reference types can be altered.
Assignment and Copying
When a reference type is assigned to a variable or passed to a function, the reference to the object is copied, not the object itself.
let obj1 = { value: 10 };
let obj2 = obj1; // obj2 is a reference to obj1
obj2.value = 20;
console.log(obj1.value); // 20
console.log(obj2.value); // 20
1. Object
Represents a collection of properties.
let person = { name: 'Alice', age: 25 };
2. Array
Represents an ordered list of values.
let numbers = [1, 2, 3, 4, 5];
3. Function
Represents a callable block of code.
function greet() {
console.log('Hello!');
}
4. Date
Represents a single moment in time.
let now = new Date();
5. RegExp
Represents a regular expression.
let regex = /ab+c/;
Differences Between Primitive and Reference Types
- Immutability vs. Mutability:
- Primitive types are immutable; their values cannot be changed.
- Reference types are mutable; their values can be modified.
- Memory Allocation:
- Primitive types are stored directly in the variable’s memory location.
- Reference types store a reference (or address) to the actual object in memory.
- Comparison:
- Primitive values are compared by value.
- Reference values are compared by reference.
// Primitive comparison
let x = 10;
let y = 10;
console.log(x === y); // true
// Reference comparison
let objA = { value: 10 };
let objB = { value: 10 };
console.log(objA === objB); // false (different references)
- Behavior in Functions
- Passing a primitive type to a function passes a copy of the value.
- Passing a reference type to a function passes a reference to the object.
function modifyPrimitive(val) {
val = val * 2;
}
let num = 10;
modifyPrimitive(num);
console.log(num); // 10
function modifyObject(obj) {
obj.value = obj.value * 2;
}
let myObj = { value: 10 };
modifyObject(myObj);
console.log(myObj.value); // 20