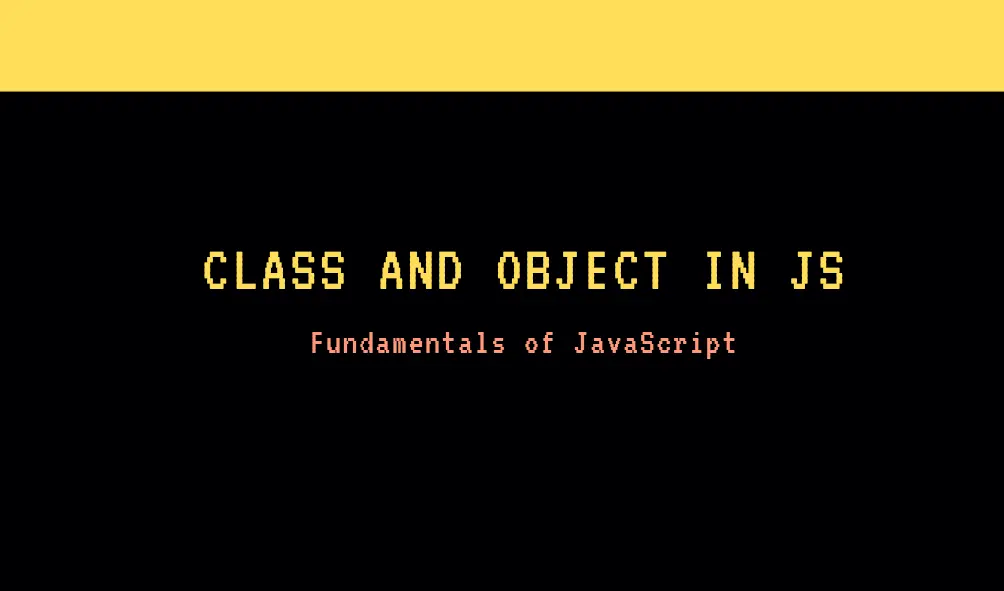
Understanding Classes and Objects in JavaScript
5. Classes And Objects
In JavaScript, objects and classes are fundamental constructs used for organizing and managing data and behavior in your code. Understanding the differences and relationships between objects and classes is crucial for effective JavaScript programming.
Objects
An object in JavaScript is a collection of properties, where a property is an association between a name (or key) and a value. These properties can hold values of primitive data types, other objects, or functions. Objects are used to represent real-world entities and are fundamental to JavaScript’s object-oriented programming (OOP) capabilities.
Creating Objects
Object Literals
let person = { name: 'John', age: 30, greet: function () { console.log('Hello, ' + this.name); }, };
Using the
Object
Constructorlet person = new Object(); person.name = 'John'; person.age = 30; person.greet = function () { console.log('Hello, ' + this.name); };
Object.create() Method
let personPrototype = { greet: function () { console.log('Hello, ' + this.name); }, }; let person = Object.create(personPrototype); person.name = 'John'; person.age = 30;
Accessing and Modifying Properties
console.log(person.name); // John
person.age = 31;
console.log(person.age); // 31
Methods
Objects can contain functions, which are called methods when they are properties of objects.
person.greet(); // Hello, John
Classes
A class in JavaScript is a blueprint for creating objects with predefined properties and methods. Classes make it easier to create multiple objects with the same structure and behavior.
Defining Classes
Class Declaration
class Person { constructor(name, age) { this.name = name; this.age = age; } greet() { console.log('Hello, ' + this.name); } }
Class Expression
let Person = class { constructor(name, age) { this.name = name; this.age = age; } greet() { console.log('Hello, ' + this.name); } };
Creating Instances
let john = new Person('John', 30);
john.greet(); // Hello, John
console.log(john.age); // 30
Inheritance
Classes can inherit from other classes using the extends
keyword. This allows for creating hierarchical class structures.
class Employee extends Person {
constructor(name, age, jobTitle) {
super(name, age); // Calls the parent class's constructor
this.jobTitle = jobTitle;
}
work() {
console.log(this.name + ' is working as a ' + this.jobTitle);
}
}
let jane = new Employee('Jane', 28, 'Software Engineer');
jane.greet(); // Hello, Jane
jane.work(); // Jane is working as a Software Engineer
Key Differences Between Objects and Classes
- Definition and Instantiation:
- Objects: Created directly using object literals,
Object
constructor, orObject.create()
. - Classes: Defined using
class
syntax and instantiated using thenew
keyword.
- Objects: Created directly using object literals,
- Inheritance:
- Objects: Inheritance is typically handled using prototypes and
Object.create()
. - Classes: Inheritance is handled using the
extends
keyword andsuper
function.
- Objects: Inheritance is typically handled using prototypes and
- Structure and Reusability:
- Objects: Often used for individual instances with specific properties and methods.
- Classes: Used to define a blueprint for creating multiple objects with the same properties and methods.
Conclusion
Understanding objects and classes in JavaScript is crucial for effective code organization and reuse. Objects provide a way to group related data and functions, while classes offer a more structured and scalable approach to creating multiple similar objects and implementing inheritance. Using these constructs appropriately allows for cleaner, more maintainable, and more robust JavaScript applications.