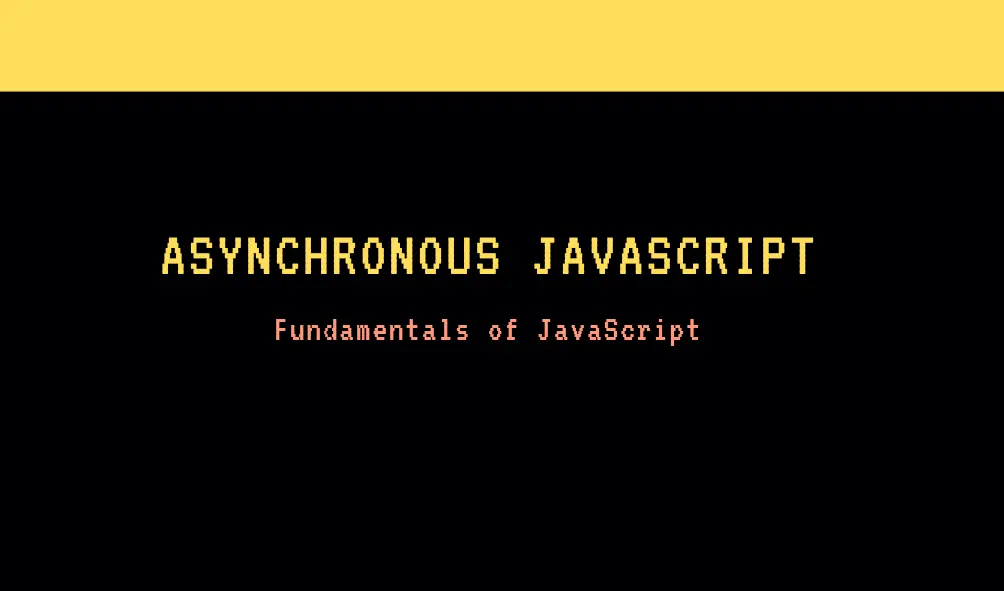
Understanding Asynchronous JavaScript
9. Asynchronous JavaScript
Asynchronous JavaScript refers to the execution of code that does not occur in a sequential manner, allowing programs to perform non-blocking operations such as network requests, file system operations, or timers. Asynchronous programming is essential for building responsive and efficient web applications, especially when dealing with tasks that may take time to complete.
Synchronous vs Asynchronous
In synchronous programming, code is executed line by line, and each line must complete before moving on to the next. This can lead to blocking behavior, where certain operations pause the execution of subsequent code until they are completed.
In asynchronous programming, tasks are executed independently of the main program flow. This allows multiple tasks to be performed simultaneously, and the program does not have to wait for each task to complete before moving on to the next.
Asynchronous Patterns in JavaScript
- Callbacks:
- Callbacks are functions passed as arguments to other functions, which are then invoked after the completion of an asynchronous operation.
- Callbacks are a common way to handle asynchronous tasks in JavaScript, but they can lead to callback hell (nested and hard-to-read code) when dealing with multiple asynchronous operations
- Promises:
- Promises provide a cleaner way to handle asynchronous operations and avoid callback hell.
- A promise represents a value that may be available now, in the future, or never.
- Promises can be in one of three states: pending, fulfilled (resolved), or rejected.
- Promises have
then()
andcatch()
methods for handling successful and failed outcomes, respectively.
- Async/Await:
- Async functions allow you to write asynchronous code that looks synchronous.
- Async functions return a promise, and you can use the
await
keyword inside them to pause execution until a promise settles. - Async functions provide a more readable and maintainable way to write asynchronous code compared to promises and callbacks.
Example: Asynchronous Code with Promises
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = 'Async Data';
if (data) {
resolve(data);
} else {
reject('Error: Data not found');
}
}, 2000);
});
}
fetchData()
.then((data) => {
console.log('Data:', data);
})
.catch((error) => {
console.error('Error:', error);
});
Example: Asynchronous Code with Async/Await
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = 'Async Data';
if (data) {
resolve(data);
} else {
reject('Error: Data not found');
}
}, 2000);
});
}
async function fetchDataAsync() {
try {
const data = await fetchData();
console.log('Data:', data);
} catch (error) {
console.error('Error:', error);
}
}
fetchDataAsync();
Common Use Cases for Asynchronous JavaScript
- HTTP Requests: Fetching data from APIs or making AJAX requests.
- File I/O: Reading from or writing to files on the server or client.
const fs = require('fs');
// Write to a file
fs.writeFile('example.txt', 'Hello, world!', (err) => {
if (err) {
console.error('Error writing to file:', err);
return;
}
console.log('File written successfully');
});
// Read from a file
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File content:', data);
});
- Timers: Executing code after a certain delay or at specified intervals.
// setTimeout example
setTimeout(() => {
console.log('Timer callback executed after 2 seconds');
}, 2000);
// setInterval example
let counter = 0;
let intervalId = setInterval(() => {
counter++;
console.log('Counter:', counter);
if (counter === 5) {
clearInterval(intervalId);
console.log('Interval stopped after 5 iterations');
}
}, 1000);
- Event Handlers: Handling user interactions such as button clicks or form submissions.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Event Handler Example</title>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
document.getElementById('myButton').addEventListener('click', function() {
console.log('Button clicked!');
});
</script>
</body>
</html>
- Promises and Callbacks: Handling asynchronous code in a clean and manageable way.
Conclusion
Asynchronous JavaScript is a fundamental concept for building modern web applications. It allows developers to write code that can handle time-consuming operations efficiently, improving the responsiveness and user experience of web applications. Understanding asynchronous patterns and mastering tools like promises and async/await is essential for writing clean, maintainable, and performant JavaScript code.