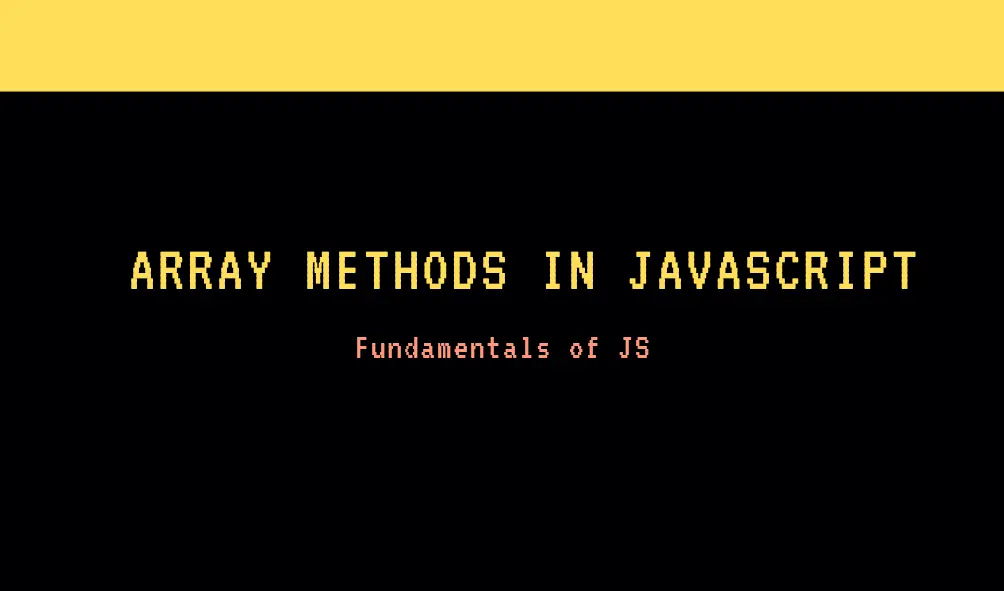
Comprehensive Guide to Array Methods in JavaScript
4. Array Methods
A. Array Creation
Array.of()
Creates a new Array instance with a variable number of arguments, regardless of number or type.
let arr = Array.of(1, 2, 3);
Array.from()
Creates a new Array instance from an array-like or iterable object.
let str = 'hello';
let arr = Array.from(str); // ['h', 'e', 'l', 'l', 'o']
B. Adding and Removing Elements
push()
Adds one or more elements to the end of an array and returns the new length.
let arr = [1, 2, 3];
arr.push(4); // [1, 2, 3, 4]
pop()
Removes the last element from an array and returns that element.
let arr = [1, 2, 3];
arr.pop(); // [1, 2]
unshift()
Adds one or more elements to the beginning of an array and returns the new length.
let arr = [1, 2, 3];
arr.unshift(0); // [0, 1, 2, 3]
shift()
Removes the first element from an array and returns that element.
let arr = [1, 2, 3];
arr.shift(); // [2, 3]
C. Accessing and Modifying Elements
concat()
Merges two or more arrays and returns a new array.
let arr1 = [1, 2];
let arr2 = [3, 4];
let newArr = arr1.concat(arr2); // [1, 2, 3, 4]
slice()
Returns a shallow copy of a portion of an array into a new array.
let arr = [1, 2, 3, 4, 5];
let newArr = arr.slice(1, 3); // [2, 3]
splice()
Adds/Removes elements from an array and returns the removed elements.
let arr = [1, 2, 3, 4];
arr.splice(2, 1, 'a', 'b'); // [1, 2, 'a', 'b', 4]
The splice()
method in JavaScript is a versatile function used to add, remove, or replace elements in an array. It modifies the original array and can be used in a variety of ways to manipulate array contents.
Syntax
array.splice(start, deleteCount, item1, item2, ...)
The splice()
method returns an array containing the deleted elements, if any.
Examples
1. Removing Elements
let fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry'];
let removed = fruits.splice(1, 2);
console.log(fruits); // ['apple', 'date', 'elderberry']
console.log(removed); // ['banana', 'cherry']
In this example:
fruits.splice(1, 2)
removes 2 elements starting from index 1.fruits
is modified to['apple', 'date', 'elderberry']
.- The removed elements
['banana', 'cherry']
are returned.
2. Adding Elements
let fruits = ['apple', 'banana', 'cherry'];
fruits.splice(2, 0, 'date', 'elderberry');
console.log(fruits); // ['apple', 'banana', 'date', 'elderberry', 'cherry']
In this example:
fruits.splice(2, 0, 'date', 'elderberry')
adds ‘date’ and ‘elderberry’ at index 2 without removing any elements.fruits
is modified to['apple', 'banana', 'date', 'elderberry', 'cherry']
.
3. Replacing Elements
let fruits = ['apple', 'banana', 'cherry'];
fruits.splice(1, 1, 'date', 'elderberry');
console.log(fruits); // ['apple', 'date', 'elderberry', 'cherry']
In this example:
fruits.splice(1, 1, 'date', 'elderberry')
removes 1 element at index 1 (‘banana’) and inserts ‘date’ and ‘elderberry’.fruits
is modified to['apple', 'date', 'elderberry', 'cherry']
.
💡 Negative indexes in splice and slice methods is used for counting from the end of an array