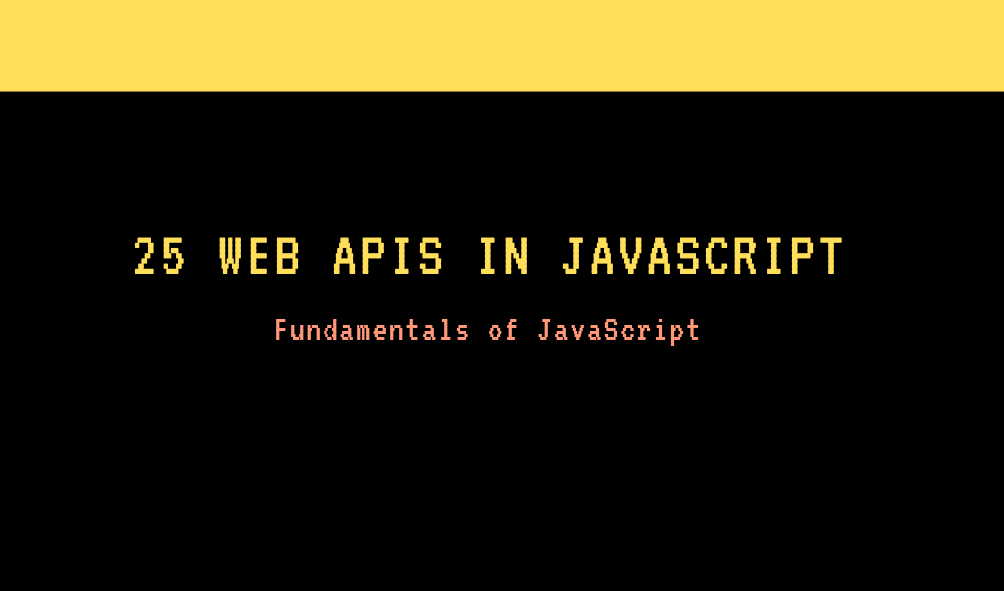
25 Essential Web APIs in JavaScript
13. Get to Know 25 Web APIs
1. Document Object Model (DOM) API
- Purpose: Represents the structure of HTML documents as a tree of objects, allowing JavaScript to interact with and manipulate the document dynamically.
- Example:
<!doctype html>
<html>
<head>
<title>DOM Example</title>
</head>
<body>
<div id="container">
<h1>Hello, DOM!</h1>
<p>This is a paragraph.</p>
</div>
<script>
// Accessing elements and modifying content
const heading = document.querySelector('h1');
heading.textContent = 'Manipulated Heading';
// Creating and appending elements
const newParagraph = document.createElement('p');
newParagraph.textContent = 'This is a new paragraph.';
document.getElementById('container').appendChild(newParagraph);
</script>
</body>
</html>
2. Fetch API
- Purpose: Provides an interface for fetching resources asynchronously across the network.
- Example:
fetch('https://api.example.com/data')
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error('Fetch error:', error));
3. Web Storage API (localStorage and sessionStorage)
- Purpose: Allows web applications to store key-value pairs locally in the browser.
- Example:
// Storing data in localStorage
localStorage.setItem('username', 'John');
// Retrieving data from localStorage
const username = localStorage.getItem('username');
console.log('Username:', username);
4. XMLHttpRequest (XHR) API
- Purpose: Provides the ability to make HTTP requests from JavaScript, allowing for AJAX-style interactions with the server.
- Example:
const xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data', true);
xhr.onload = function () {
if (xhr.status === 200) {
console.log('Data:', xhr.responseText);
} else {
console.error('XHR error:', xhr.statusText);
}
};
xhr.onerror = function () {
console.error('XHR network error');
};
xhr.send();
5. Canvas API
- Purpose: Provides a way to draw graphics, animations, and other visual effects on a web page using JavaScript.
- Example:
<canvas id="myCanvas" width="200" height="100"></canvas>
<script>
const canvas = document.getElementById('myCanvas');
const context = canvas.getContext('2d');
context.fillStyle = 'blue';
context.fillRect(10, 10, 150, 80);
</script>
6. Geolocation API
- Purpose: Allows web applications to retrieve the user’s geographical location information.
- Example:
if ('geolocation' in navigator) {
navigator.geolocation.getCurrentPosition(
(position) => {
console.log('Latitude:', position.coords.latitude);
console.log('Longitude:', position.coords.longitude);
},
(error) => {
console.error('Geolocation error:', error);
}
);
} else {
console.error('Geolocation not supported');
}
7. Web Audio API
- Purpose: Provides capabilities for playing and manipulating audio in web applications.
- Example:
const audioContext = new AudioContext();
const oscillator = audioContext.createOscillator();
oscillator.frequency.setValueAtTime(440, audioContext.currentTime);
oscillator.connect(audioContext.destination);
oscillator.start();
8. Web Workers API
- Purpose: Allows running scripts in background threads to perform tasks without blocking the main execution thread.
- Example:
// main.js
const worker = new Worker('worker.js');
worker.postMessage('Hello from main thread');
worker.onmessage = (event) => {
console.log('Message from worker:', event.data);
};
// worker.js
self.onmessage = (event) => {
console.log('Message from main thread:', event.data);
self.postMessage('Hello from worker');
};
9. IndexedDB API
- Purpose: Provides a way to store large amounts of structured data persistently in the browser.
- Example:
const request = indexedDB.open('myDatabase', 1);
request.onerror = (event) => {
console.error('IndexedDB error:', event.target.error);
};
request.onsuccess = (event) => {
const db = event.target.result;
const transaction = db.transaction('storeName', 'readwrite');
const store = transaction.objectStore('storeName');
store.put({ id: 1, name: 'John Doe' });
};
10. Notification API
- Purpose: Allows web applications to display notifications to the user outside of the web page.
- Example:
if ('Notification' in window) {
Notification.requestPermission().then((permission) => {
if (permission === 'granted') {
new Notification('Hello, world!');
}
});
} else {
console.error('Notification not supported');
}
These are just a few examples of commonly used Web APIs in JavaScript. Each API serves a specific purpose and provides developers with powerful tools for building modern web applications.
11. Web Speech API
- Purpose: Provides speech recognition (SpeechRecognition) and speech synthesis (SpeechSynthesis) capabilities in web applications.
- Example (Speech Recognition):
const recognition = new SpeechRecognition();
recognition.onresult = (event) => {
const transcript = event.results[0][0].transcript;
console.log('Speech recognized:', transcript);
};
recognition.start();
12. WebRTC API
- Purpose: Enables real-time communication (voice, video, and data) between browsers.
- Example:
const peerConnection = new RTCPeerConnection();
navigator.mediaDevices
.getUserMedia({ audio: true, video: true })
.then((stream) => {
stream.getTracks().forEach((track) => peerConnection.addTrack(track, stream));
})
.catch((error) => {
console.error('MediaDevices error:', error);
});
13. Intersection Observer API
- Purpose: Allows developers to observe changes in the intersection of an element with an ancestor element or the viewport.
- Example:
const observer = new IntersectionObserver((entries) => {
entries.forEach((entry) => {
if (entry.isIntersecting) {
console.log('Element is visible');
} else {
console.log('Element is not visible');
}
});
});
observer.observe(document.querySelector('#targetElement'));
14. File API (FileReader, Blob)
- Purpose: Provides methods for reading files from the user’s local file system and working with file data in web applications.
- Example (FileReader):
<input type="file" id="fileInput" />
<script>
const fileInput = document.getElementById('fileInput');
fileInput.addEventListener('change', (event) => {
const file = event.target.files[0];
const reader = new FileReader();
reader.onload = () => {
console.log('File content:', reader.result);
};
reader.readAsText(file);
});
</script>
15. Battery Status API
- Purpose: Allows web applications to access information about the device’s battery status and level.
- Example:
navigator.getBattery().then((battery) => {
console.log('Battery level:', battery.level * 100 + '%');
console.log('Charging:', battery.charging);
});
16. Mutation Observer API
- Purpose: Provides a way to observe changes to the DOM and receive notifications when mutations occur.
- Example:
const observer = new MutationObserver((mutations) => {
mutations.forEach((mutation) => {
console.log('Mutation type:', mutation.type);
console.log('Target node:', mutation.target);
});
});
const config = { attributes: true, childList: true, subtree: true };
observer.observe(document.body, config);
17. Web Animations API
- Purpose: Allows developers to create and control animations in web applications using JavaScript.
- Example:
const element = document.getElementById('animatedElement');
element.animate([{ transform: 'translateX(0px)' }, { transform: 'translateX(100px)' }], {
duration: 1000,
iterations: Infinity,
});
18. Clipboard API
- Purpose: Provides asynchronous access to the system clipboard, allowing web applications to read from and write to it.
- Example:
navigator.clipboard
.writeText('Hello, clipboard!')
.then(() => {
console.log('Text copied to clipboard');
})
.catch((error) => {
console.error('Clipboard write error:', error);
});
19. Web Cryptography API
- Purpose: Provides cryptographic functionalities such as hashing, encryption, and decryption in web applications.
- Example (Hashing):
crypto.subtle
.digest('SHA-256', new TextEncoder().encode('hello'))
.then((hash) => {
console.log('Hash:', hex(hash));
})
.catch((error) => {
console.error('Hashing error:', error);
});
20. Web Bluetooth API
- Purpose: Allows web applications to communicate with Bluetooth devices nearby.
- Example (Scanning for Devices):
navigator.bluetooth
.requestDevice({ filters: [{ services: ['heart_rate'] }] })
.then((device) => {
console.log('Bluetooth device:', device);
})
.catch((error) => {
console.error('Bluetooth error:', error);
});
21. Web Speech API (SpeechSynthesis)
- Purpose: Allows web applications to generate synthesized speech from text data.
- Example:
const utterance = new SpeechSynthesisUtterance('Hello, world!');
speechSynthesis.speak(utterance);
22. Payment Request API
- Purpose: Allows web applications to request payment information from users, facilitating seamless and secure online payments.
- Example:
const paymentRequest = new PaymentRequest(methodData, details, options);
paymentRequest
.show()
.then((paymentResponse) => {
console.log('Payment response:', paymentResponse);
return paymentResponse.complete();
})
.catch((error) => {
console.error('Payment error:', error);
});
23. Pointer Lock API
- Purpose: Provides a way to lock the mouse pointer within the boundaries of a specified element, useful for games and immersive experiences.
- Example:
const element = document.getElementById('lockedElement');
element.requestPointerLock();
24. Web Authentication API (WebAuthn)
- Purpose: Allows web applications to authenticate users using strong cryptographic credentials, such as biometrics or hardware tokens.
- Example (Registration):
navigator.credentials.create({
publicKey: {
rp: { name: 'Example Corp' },
user: { id: new Uint8Array(16), name: 'user@example.com', displayName: 'User' },
challenge: new Uint8Array(32),
pubKeyCredParams: [{ type: 'public-key', alg: -7 }],
timeout: 60000,
attestation: 'direct',
},
});